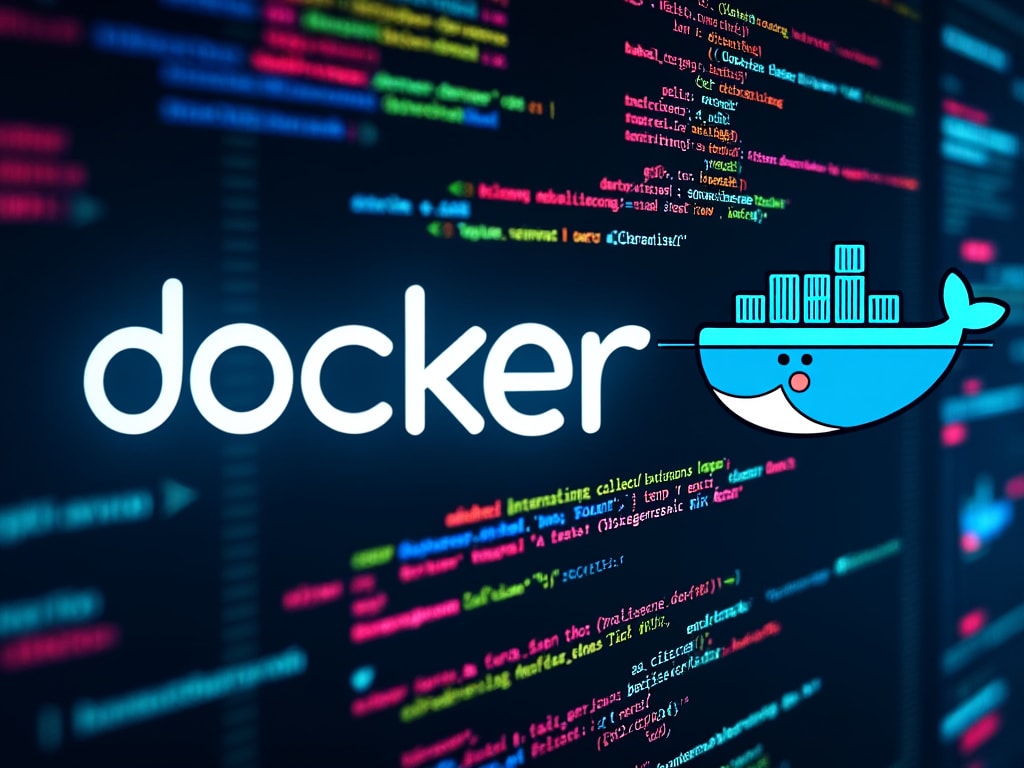
Programming
This will tell you how to create a Docker Image and put it on docker up, and then transferring to a server.
Creating a better Docker Container for Payload/Next.JS
In a previous post I showed you how to create a docker container and deploy it, however this is a much better way. This way allows you to upload less of your code, and deploy the docker container only using the files necessary. This also passes all the env fields during build
First create a new Dockerfile deleting any old docker files
1# Base image for all stages2FROM node:22-alpine AS base34# Common ARGs for build-time variables (Make them available for all stages)5ARG DATABASE_URI6ARG PAYLOAD_SECRET7ARG PAYLOAD_PUBLIC_SERVER_URL8ARG NEXT_PUBLIC_SERVER_URL9ARG NEXT_PUBLIC_IS_LIVE10ARG PAYLOAD_PUBLIC_DRAFT_SECRET11ARG NEXT_PRIVATE_DRAFT_SECRET12ARG REVALIDATION_KEY13ARG NEXT_PRIVATE_REVALIDATION_KEY1415# ENV variables (Optional: persist build-time variables as runtime ENV)16ENV DATABASE_URI=${DATABASE_URI}17ENV PAYLOAD_SECRET=${PAYLOAD_SECRET}18ENV PAYLOAD_PUBLIC_SERVER_URL=${PAYLOAD_PUBLIC_SERVER_URL}19ENV NEXT_PUBLIC_SERVER_URL=${NEXT_PUBLIC_SERVER_URL}20ENV NEXT_PUBLIC_IS_LIVE=${NEXT_PUBLIC_IS_LIVE}21ENV PAYLOAD_PUBLIC_DRAFT_SECRET=${PAYLOAD_PUBLIC_DRAFT_SECRET}22ENV NEXT_PRIVATE_DRAFT_SECRET=${NEXT_PRIVATE_DRAFT_SECRET}23ENV REVALIDATION_KEY=${REVALIDATION_KEY}24ENV NEXT_PRIVATE_REVALIDATION_KEY=${NEXT_PRIVATE_REVALIDATION_KEY}2526# Dependencies Stage27FROM base AS deps28RUN apk add --no-cache libc6-compat29WORKDIR /app3031COPY package.json yarn.lock* package-lock.json* pnpm-lock.yaml* ./3233RUN \34 if [ -f yarn.lock ]; then yarn --frozen-lockfile; \35 elif [ -f package-lock.json ]; then npm ci; \36 elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm i --frozen-lockfile; \37 else echo "Lockfile not found." && exit 1; \38 fi3940# Build Stage41FROM base AS builder42WORKDIR /app43COPY --from=deps /app/node_modules ./node_modules44COPY . .4546# Use build-time variables during build47RUN \48 if [ -f yarn.lock ]; then yarn run build; \49 elif [ -f package-lock.json ]; then npm run build; \50 elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm run build; \51 else echo "Lockfile not found." && exit 1; \52 fi5354# Runner Stage55FROM base AS runner56WORKDIR /app5758# Use runtime variables here59ENV NODE_ENV production60ENV NEXT_TELEMETRY_DISABLED 16162# Create nextjs user and group63RUN addgroup --system --gid 1001 nodejs64RUN adduser --system --uid 1001 nextjs6566COPY --from=builder /app/public ./public6768RUN mkdir .next6970COPY --from=builder --chown=nextjs:nodejs /app/.next/standalone ./71COPY --from=builder --chown=nextjs:nodejs /app/.next/static ./.next/static7273RUN chown -R nextjs:nodejs /app7475USER nextjs7677EXPOSE 30007879ENV PORT 30008081CMD HOSTNAME="0.0.0.0" node server.js82
Next create a script to build the docker:
1#!/bin/bash2export $(cat .env | grep "=" | xargs) && \3docker buildx build --platform linux/amd64,linux/arm64 \4 --build-arg DATABASE_URI=$DATABASE_URI \5 --build-arg PAYLOAD_SECRET=$PAYLOAD_SECRET \6 --build-arg PAYLOAD_PUBLIC_SERVER_URL=$PAYLOAD_PUBLIC_SERVER_URL \7 --build-arg NEXT_PUBLIC_SERVER_URL=$NEXT_PUBLIC_SERVER_URL \8 --build-arg NEXT_PUBLIC_IS_LIVE=$NEXT_PUBLIC_IS_LIVE \9 --build-arg PAYLOAD_PUBLIC_DRAFT_SECRET=$PAYLOAD_PUBLIC_DRAFT_SECRET \10 --build-arg NEXT_PRIVATE_DRAFT_SECRET=$NEXT_PRIVATE_DRAFT_SECRET \11 --build-arg REVALIDATION_KEY=$REVALIDATION_KEY \12 --build-arg NEXT_PRIVATE_REVALIDATION_KEY=$NEXT_PRIVATE_REVALIDATION_KEY \13 -t your_docker_name/appname_app:latest --push .14
The server side is done the same as my previous post on creating a docker image
Preston Garrison
This will tell you how to create a Docker Image and put it on docker up, and then transferring to a server.